serialize层
dubbo的序列化方式包括:hessian2、fastjson、fst、jdk、kryo
只要通过配置<dubbo:protocol serialization="">
或<dubbo:service serialization="">
就可以实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| @SPI("hessian2") public interface Serialization {
byte getContentTypeId();
String getContentType();
@Adaptive ObjectOutput serialize(URL url, OutputStream output) throws IOException;
@Adaptive ObjectInput deserialize(URL url, InputStream input) throws IOException;
}
|
Hessian2Serialization
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| public class Hessian2Serialization implements Serialization {
public static final byte ID = 2;
public byte getContentTypeId() { return ID; }
public String getContentType() { return "x-application/hessian2"; }
public ObjectOutput serialize(URL url, OutputStream out) throws IOException { return new Hessian2ObjectOutput(out); }
public ObjectInput deserialize(URL url, InputStream is) throws IOException { return new Hessian2ObjectInput(is); }
}
|
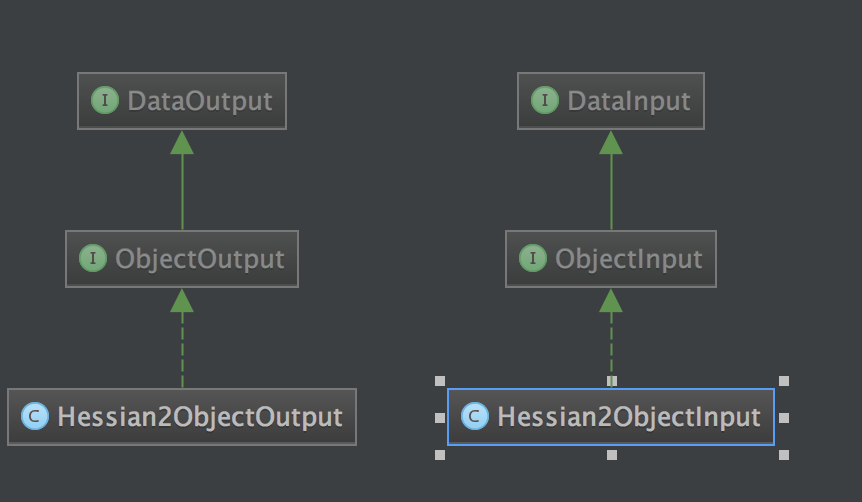
KryoSerialization
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| public class KryoSerialization implements Serialization {
public byte getContentTypeId() { return 8; }
public String getContentType() { return "x-application/kryo"; }
public ObjectOutput serialize(URL url, OutputStream out) throws IOException { return new KryoObjectOutput(out); }
public ObjectInput deserialize(URL url, InputStream is) throws IOException { return new KryoObjectInput(is); } }
|
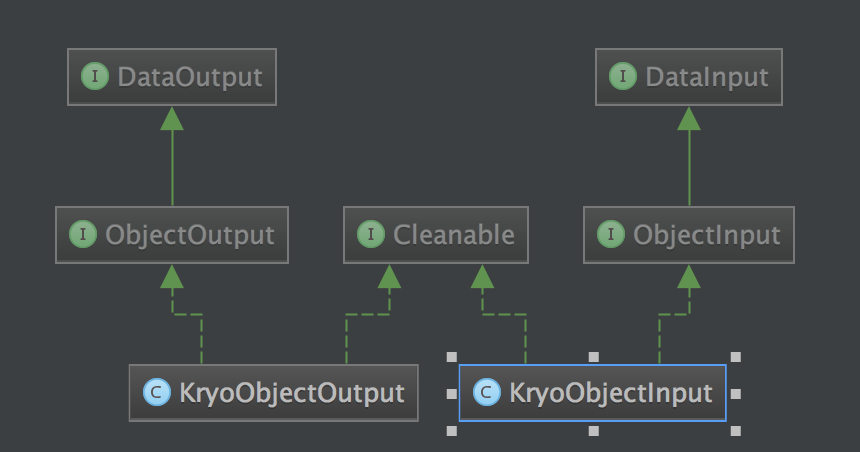
FastJsonSerialization
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| public class FastJsonSerialization implements Serialization {
public byte getContentTypeId() { return 6; }
public String getContentType() { return "text/json"; }
public ObjectOutput serialize(URL url, OutputStream output) throws IOException { return new FastJsonObjectOutput(output); }
public ObjectInput deserialize(URL url, InputStream input) throws IOException { return new FastJsonObjectInput(input); }
}
|

FstSerialization
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| public class FstSerialization implements Serialization {
public byte getContentTypeId() { return 9; }
public String getContentType() { return "x-application/fst"; }
public ObjectOutput serialize(URL url, OutputStream out) throws IOException { return new FstObjectOutput(out); }
public ObjectInput deserialize(URL url, InputStream is) throws IOException { return new FstObjectInput(is); } }
|

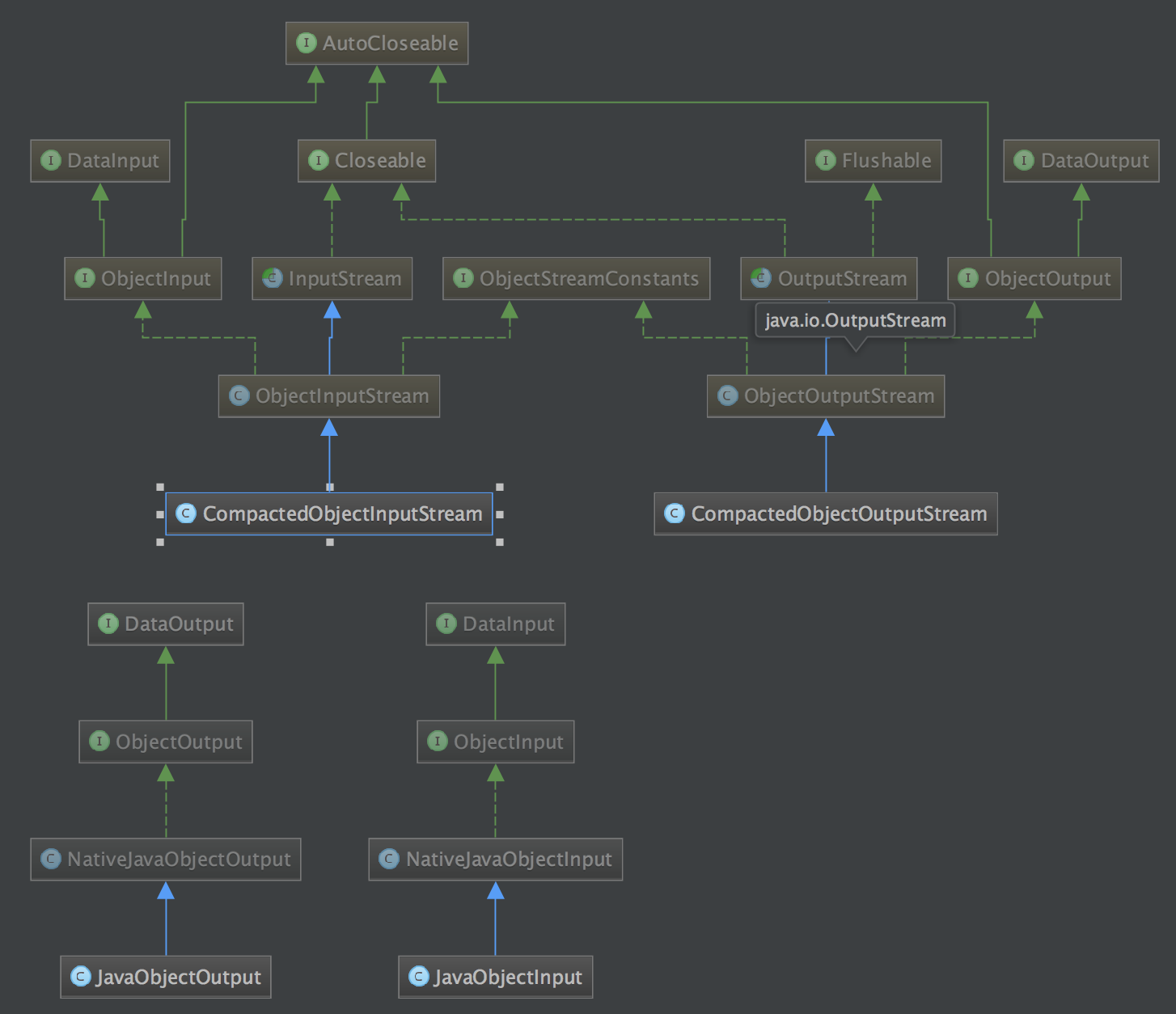
从上面我们可以看到最重要的接口就是ObjectInput、ObjectOutput、DataInput、DataOutput
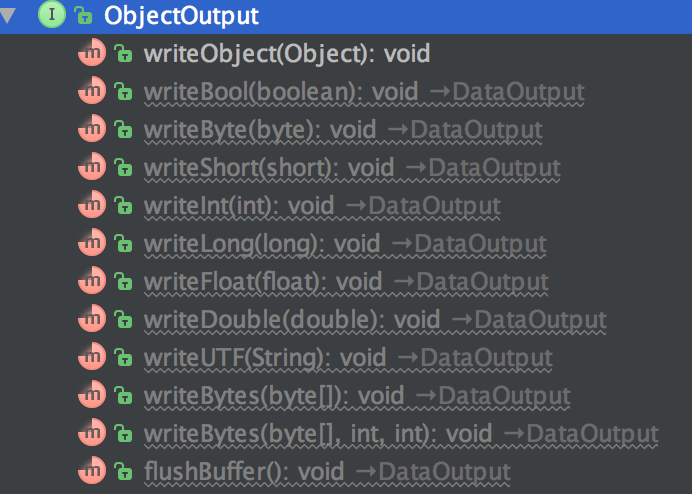
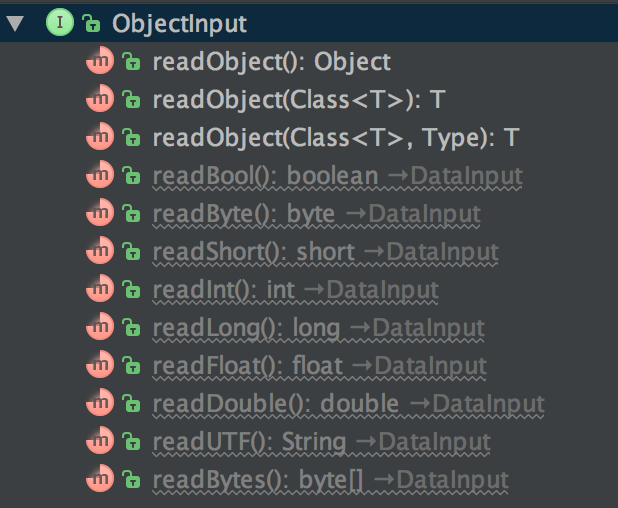